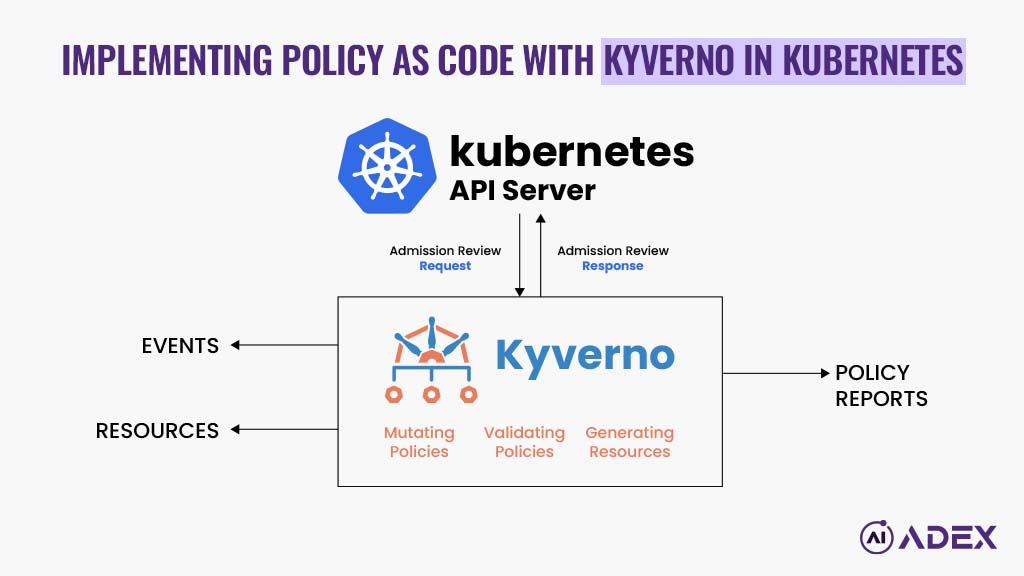
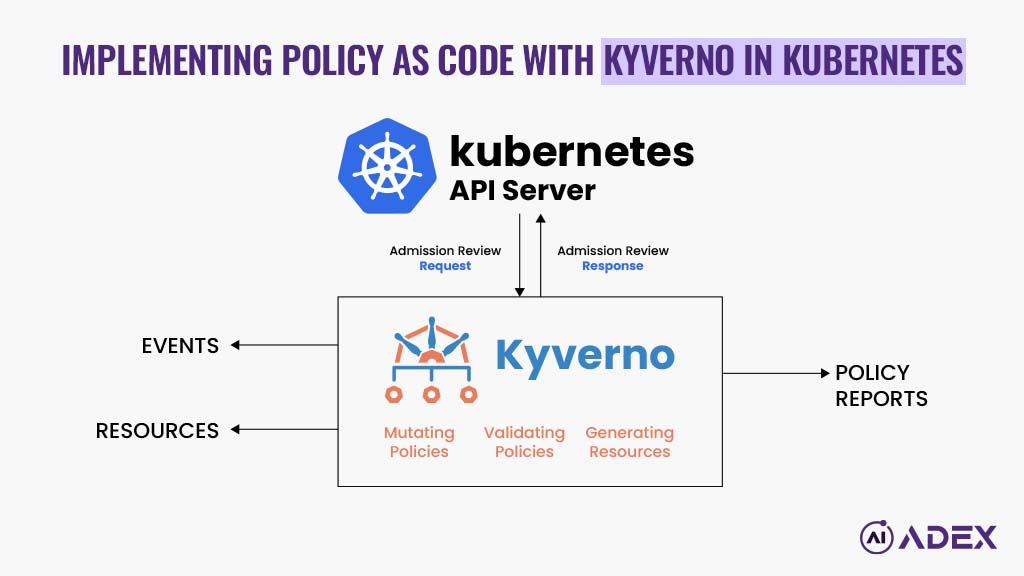
Achieving Policy as Code with Kyverno in Kubernetes
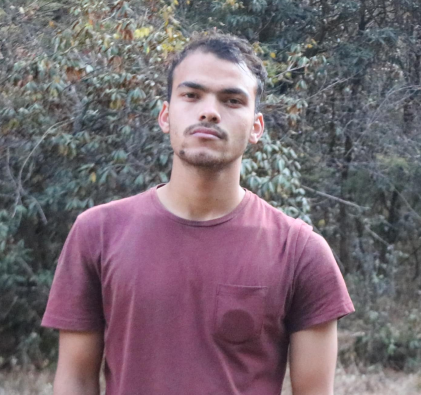
Written By Mukesh Awasthi
Jan 27, 2025
An adept DevOps Engineer with expertise in AWS cloud infrastructure, Kubernetes orchestration, monitoring, and security. With a strong focus on automating workflows, he has successfully implemented scalable CI/CD pipelines and optimized cloud-native solutions. Mukesh is committed to maintaining a secure cloud environment while leveraging cutting-edge technologies to ensure system reliability and performance across cloud platforms.
Latest Blogs
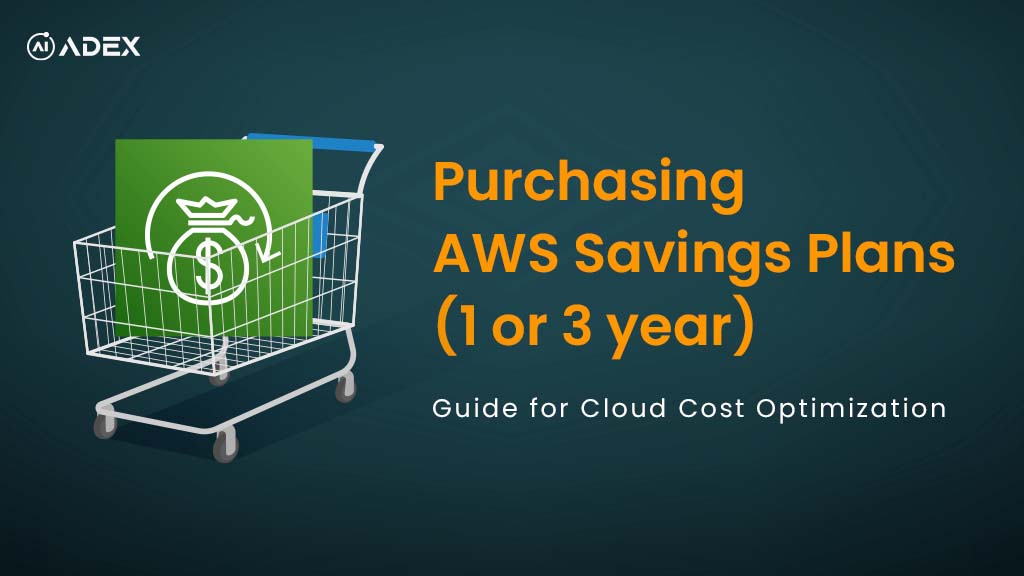
Purchasing AWS Savings Plans (1 or 3 year): Step-by-Step Guide for Cloud Cost Optimization
Managing AWS costs has become a top priority as cloud spending continues to outpace other IT expense...
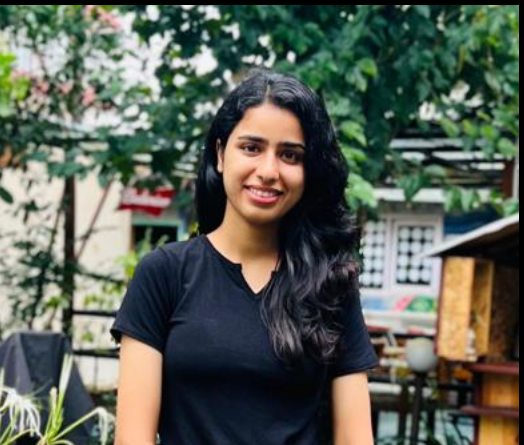
Prashansa Joshi
Jul 21, 2025
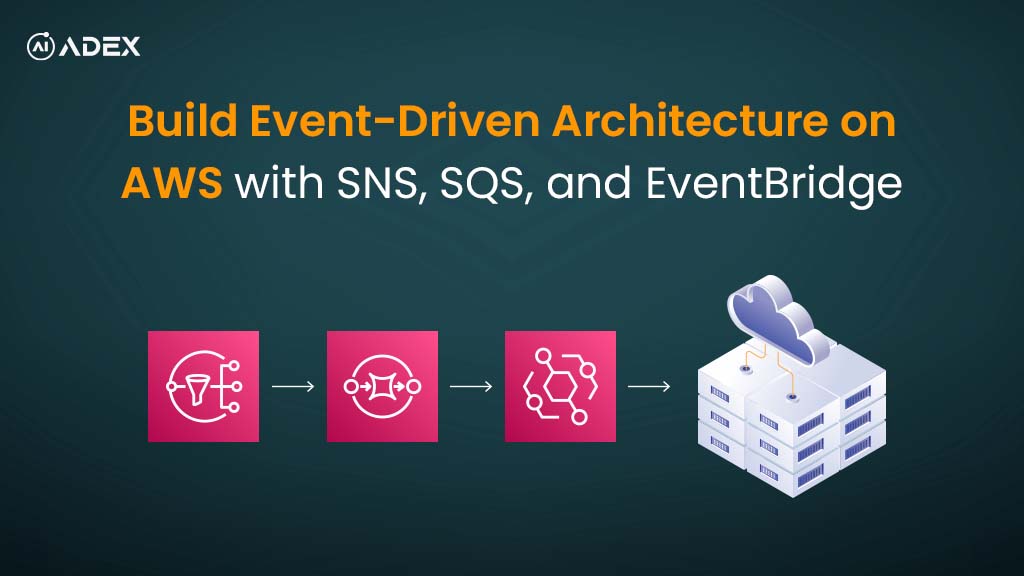
Getting Started with Event-Driven Architecture on AWS using SNS, SQS, and EventBridge
Event-driven architecture is a cornerstone of modern cloud applications, enabling systems to respond...
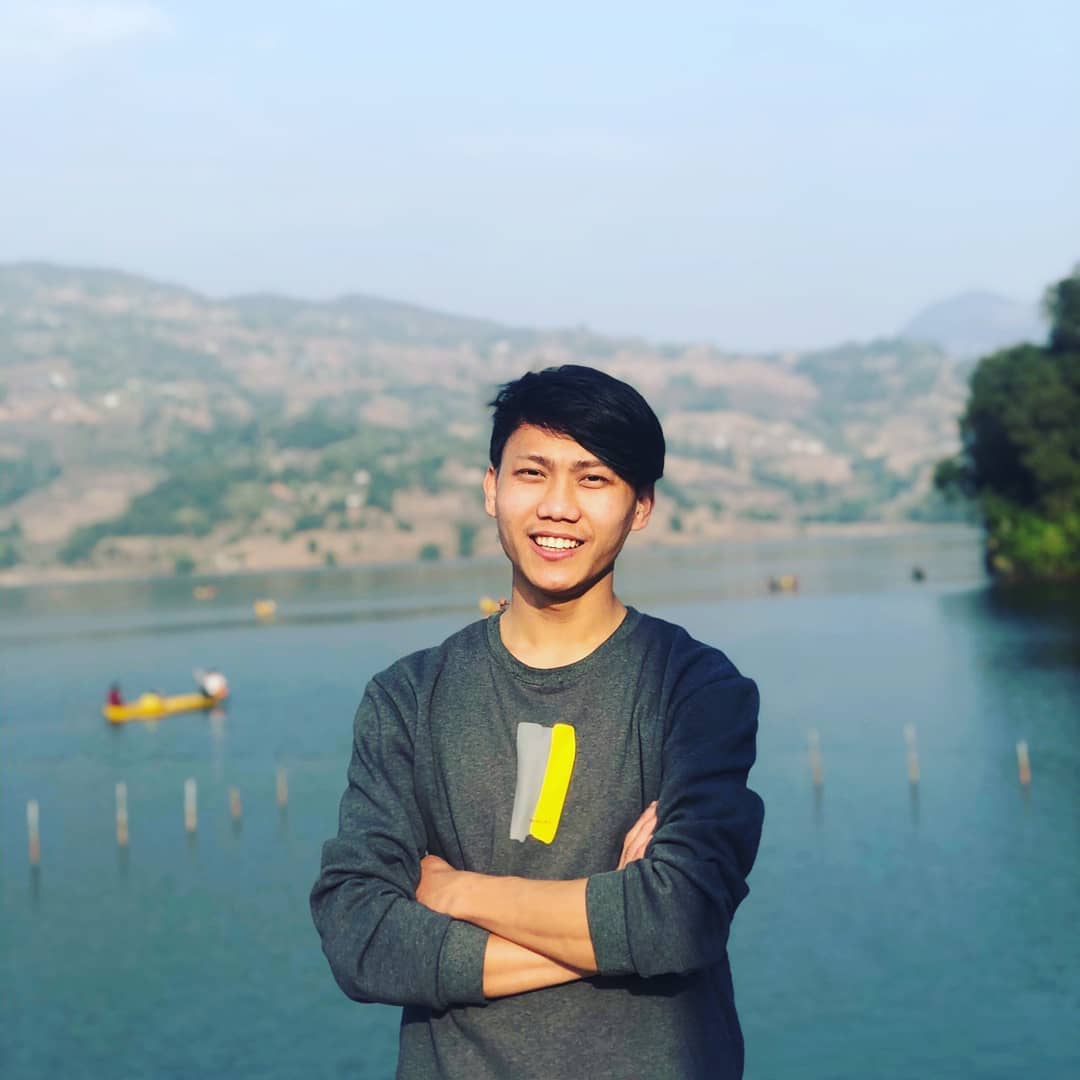
Chandra Rana
Jul 18, 2025
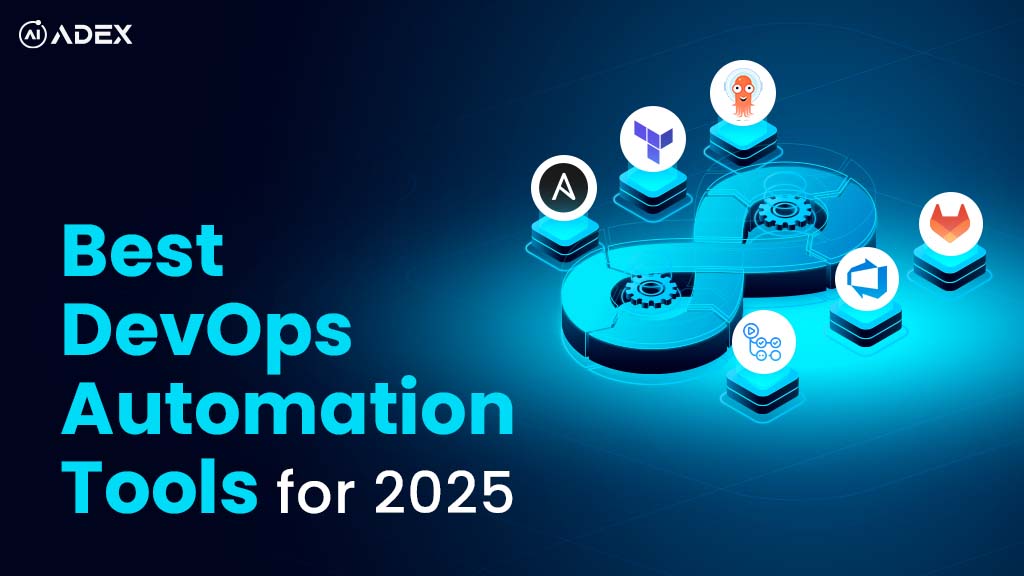
Best DevOps Automation Tools
DevOps in 2025 isn’t just about faster releases; it’s about intelligent, autonomous delivery pipelin...
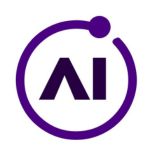
Adex International
Jul 17, 2025
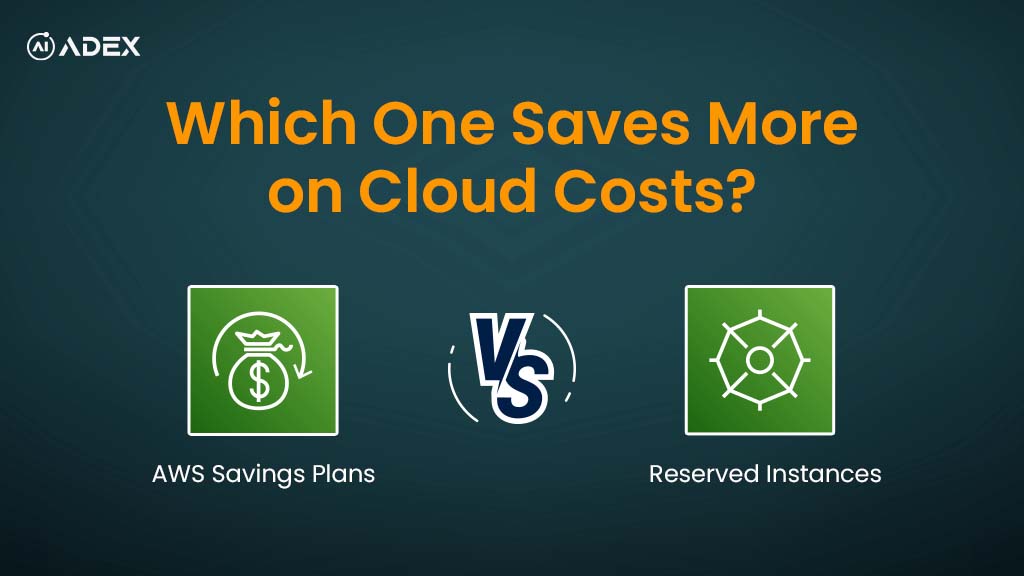
AWS Savings Plans vs. Reserved Instances: Which Saves More for Your Cloud Costs?
As more organizations utilize AWS services, they require more effective methods to manage their cost...
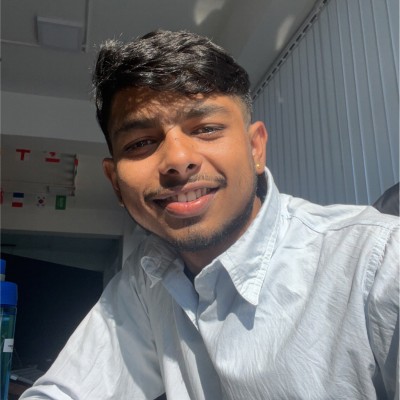
Saugat Tiwari
Jul 11, 2025
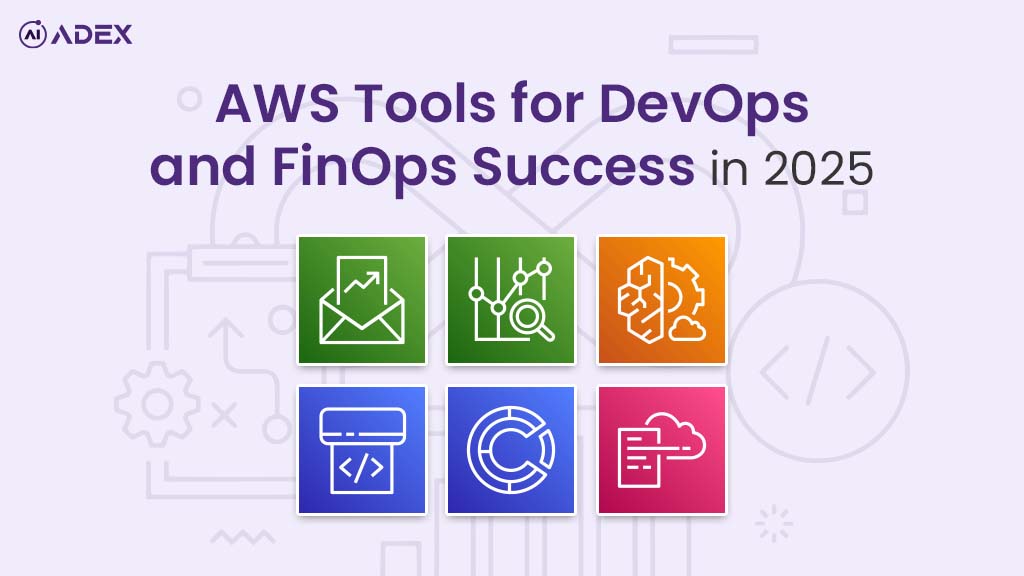
AWS Tools for DevOps and FinOps Success
As cloud environments grow more complex, success in 2025 hinges on delivering faster while spending...
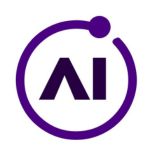
Adex International
Jul 03, 2025
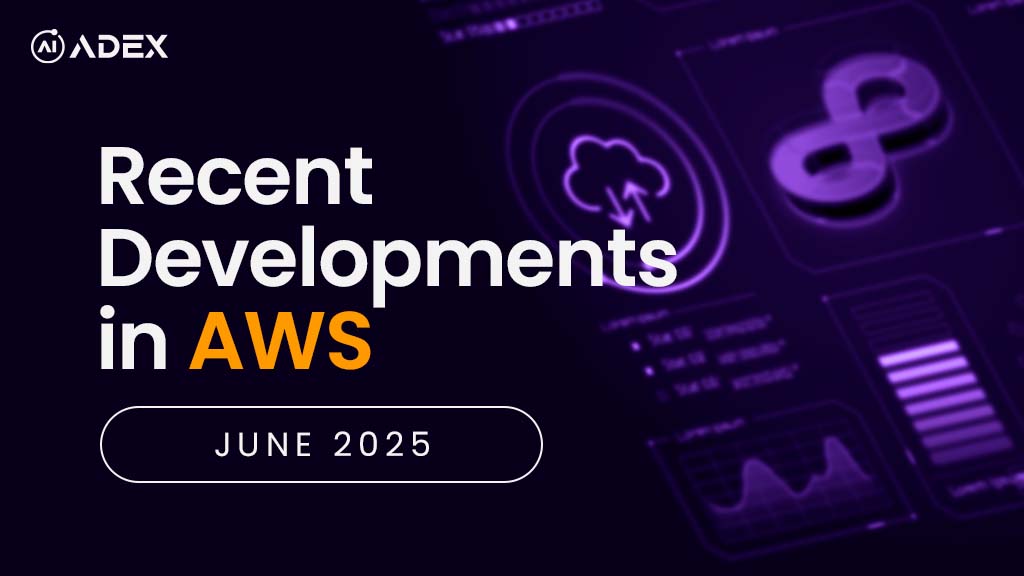
AWS Updates in June 2025: Advancements in Cloud Efficiency and Agility
AWS continued to raise the bar with its June 2025 updates, delivering a powerful mix of intelligent...
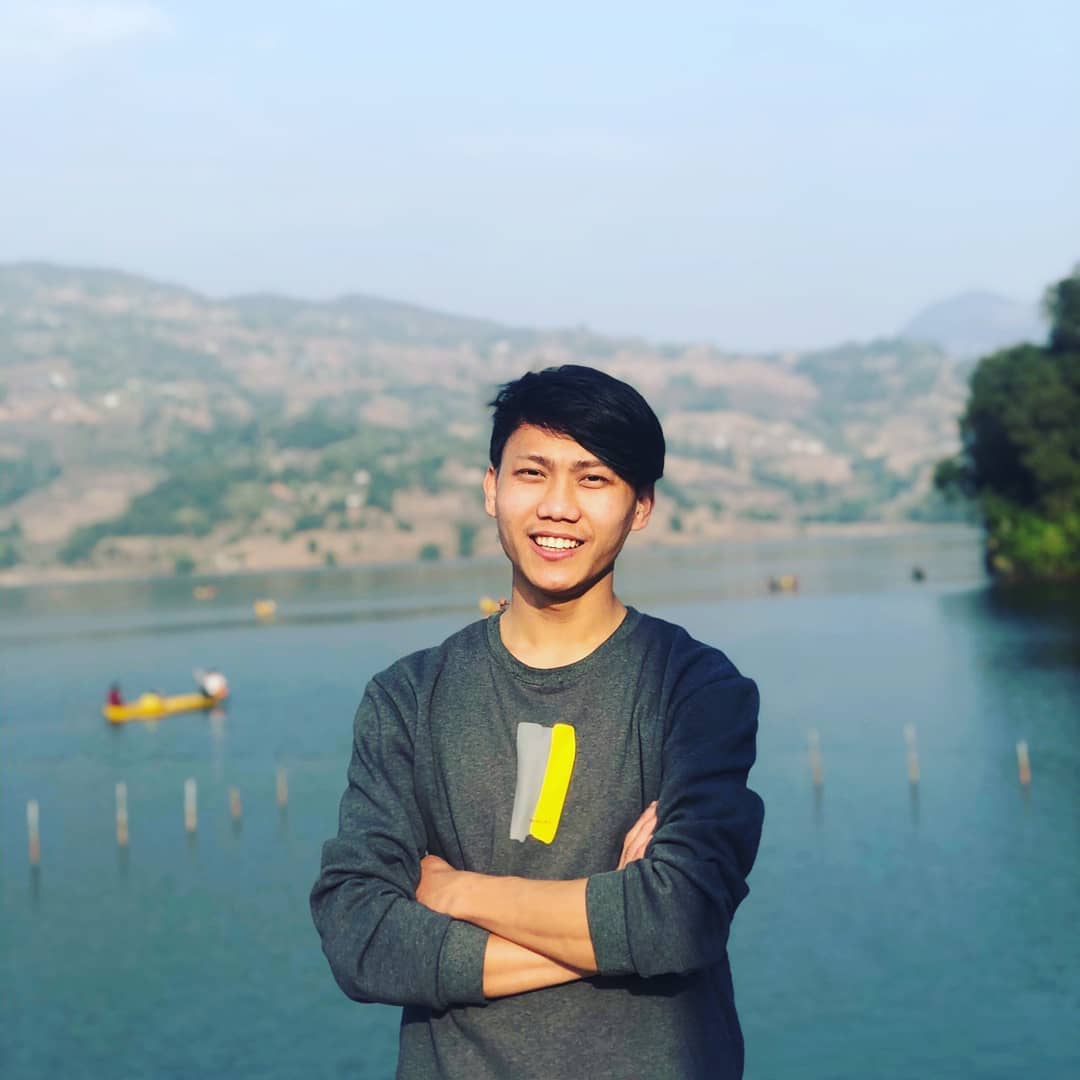
Chandra Rana
Jul 03, 2025
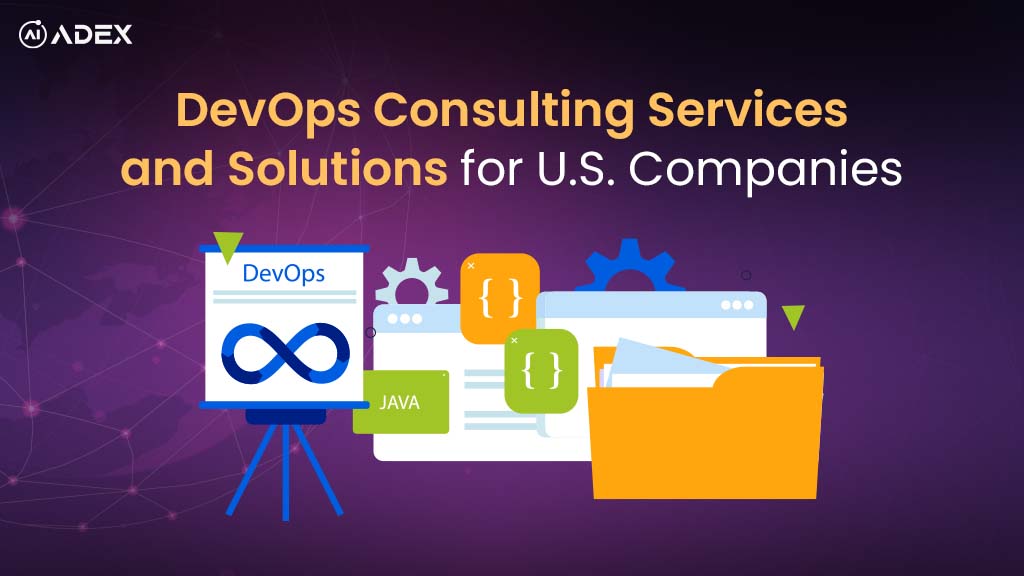
DevOps Consulting Services and Solutions for U.S. Companies (2025)
U.S. companies face growing pressure to ship software faster, modernize legacy systems, and make eve...
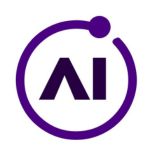
Adex International
Jun 30, 2025
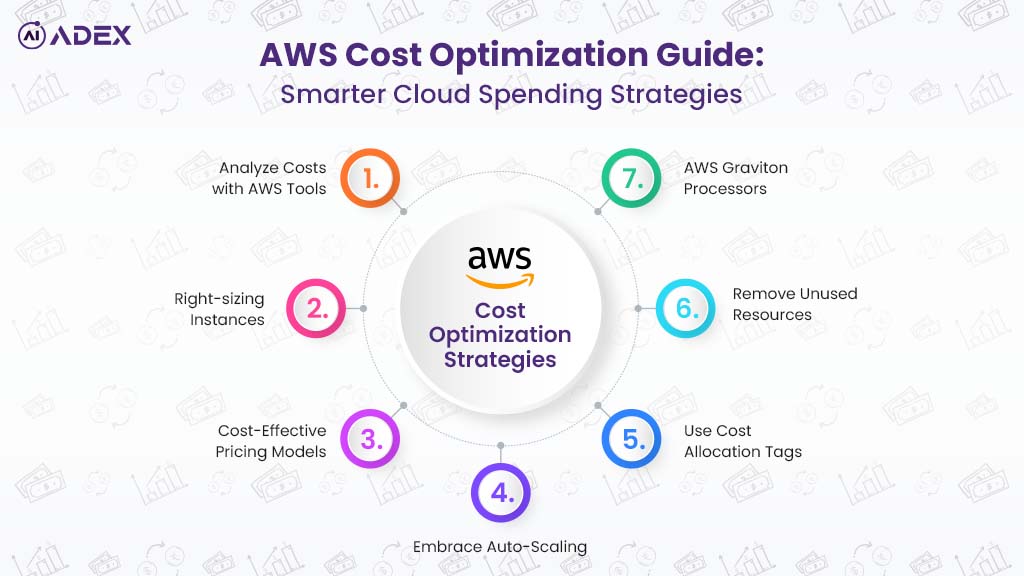
AWS Cost Optimization Guide: Smarter Cloud Spending Strategies
Amazon Web Services (AWS) powers millions of businesses worldwide with flexible, scalable cloud infr...
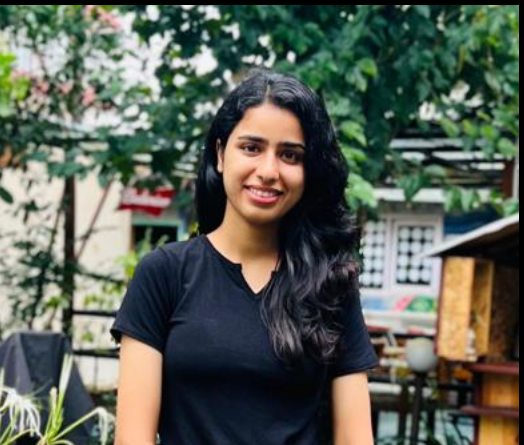
Prashansa Joshi
Jun 25, 2025
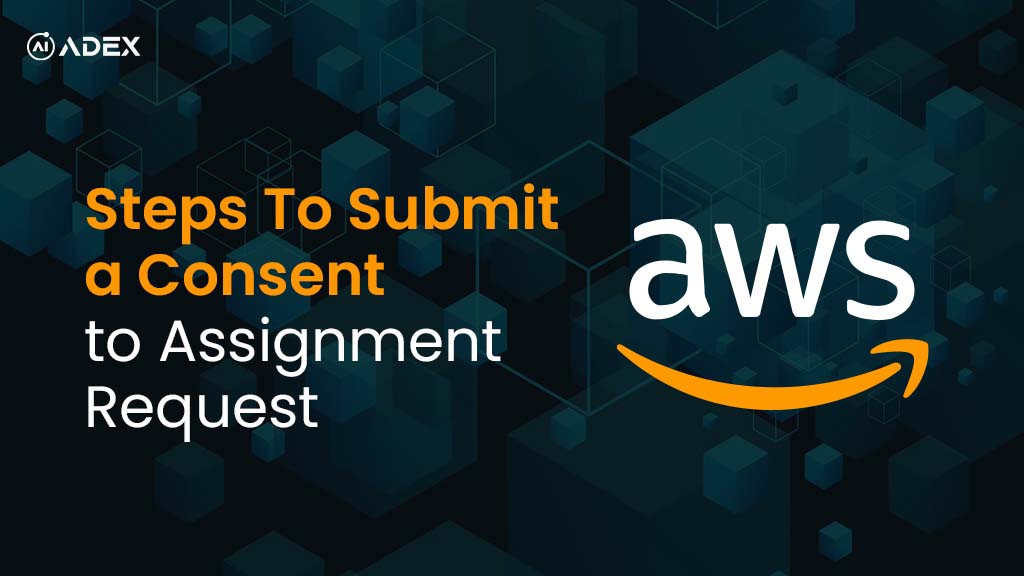
How to Submit an AWS Consent to Assignment Request
When transferring an AWS account between two legally distinct entities, such as during a merger, acq...
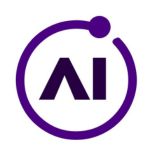
Adex International
Jun 24, 2025
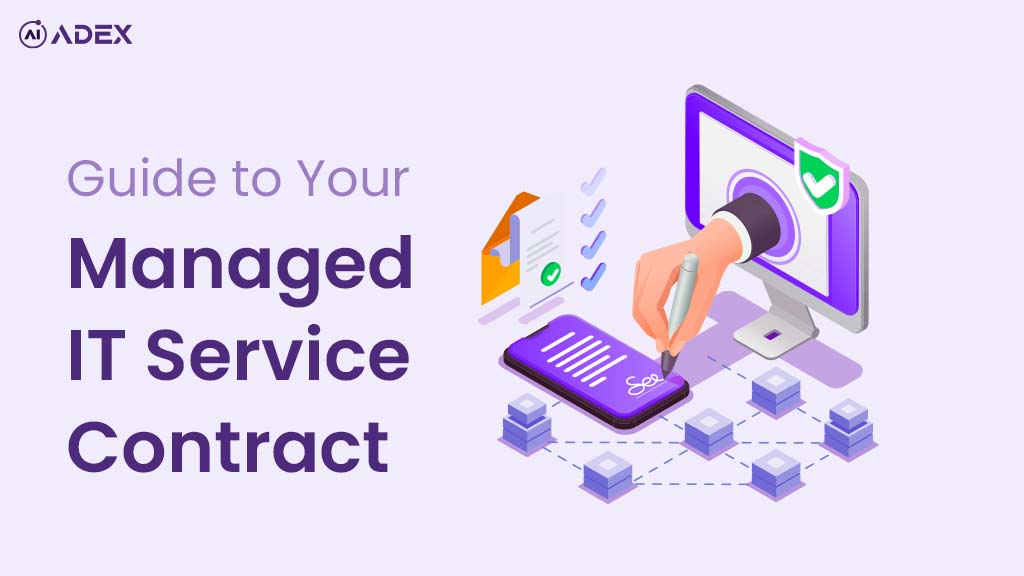
A Complete Guide to Your Managed IT Service
A well-structured Managed IT Service Contract is more than a legal technicality; it is a strategic d...
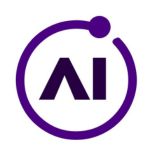
Adex International
Jun 18, 2025