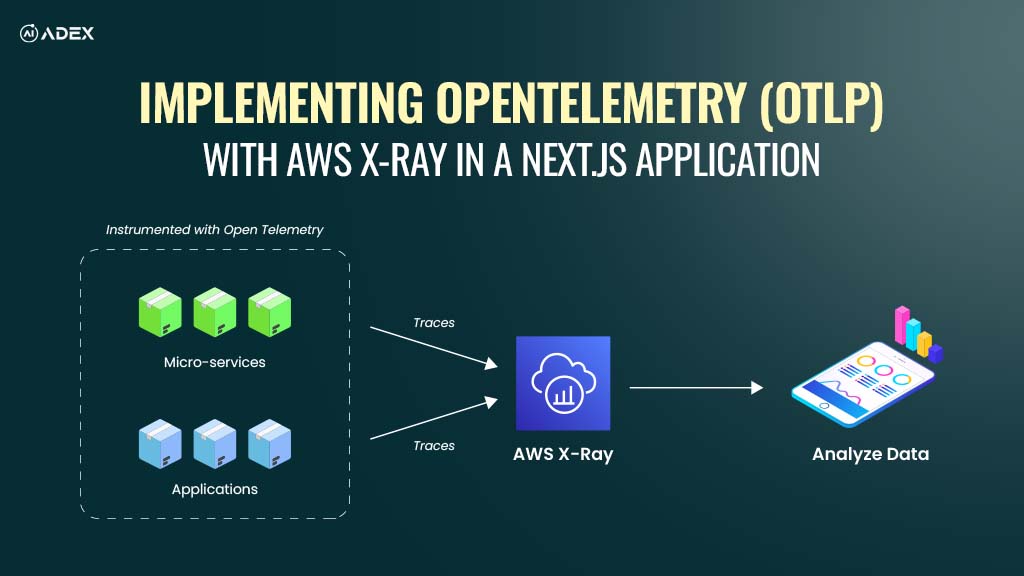
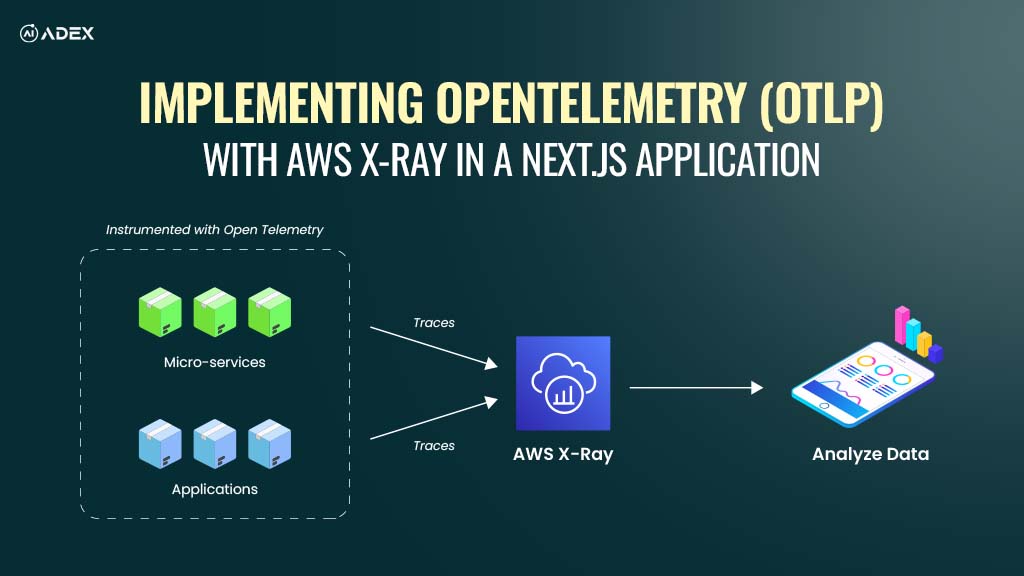
Implementing OpenTelemetry (OTEL) with AWS X-Ray in a Next.js Application
Tracing is essential for understanding request flows and diagnosing performance issues in distributed applications. Without proper tracing tools, debugging failures and identifying bottlenecks can be challenging and time-consuming.
Integrating OpenTelemetry (OTEL) with AWS X-Ray provides a structured approach to capturing and analyzing traces. This allows developers to gain deeper insights and optimize application performance.
This blog walks you through on how to implement AWS OpenTelemetry (OTEL) Collector with AWS X-Ray in a Next.js application to improve troubleshooting efficiency and system visibility.
How OpenTelemetry and AWS X-Ray Solve Tracing Challenges
OpenTelemetry (OTEL) is a set of APIs, libraries, agents, and instrumentation that enable observability through distributed tracing and metrics collection. It allows applications to send trace data to various observability backends, including AWS X-Ray.
AWS X-Ray helps analyze and debug distributed applications by tracing requests and visualizing their behavior.
When integrated with OpenTelemetry, AWS X-Ray processes the collected trace data, maps request flows across services, and identifies latency, errors, and dependencies—all within a unified observability pipeline.
For more, refer to OpenTelemetry Collector documentation and AWS X-Ray documentation.
Scenario: Challenges When Debugging a Next.js Application
Imagine a Next.js application that relies on multiple backend services, such as APIs, databases, and third-party integrations. When users experience slow page loads or intermittent errors, identifying the root cause without proper tracing becomes guesswork.
Developers end up digging through logs across different services, trying to connect scattered error messages. Debugging is time-consuming and inefficient without a clear way to track request flows.
Steps to Implement OpenTelemetry (OTEL) with AWS X-Ray
Step 1: Setting Up OpenTelemetry Collector
The OpenTelemetry Collector is a proxy that receives trace data from the Next.js application and forwards it to AWS X-Ray.
Use the following configuration in the otel-collector-config.yaml
file to define the collector's behavior:
receivers:
otlp:
protocols:
http:
endpoint: 0.0.0.0:4318
grpc:
endpoint: 0.0.0.0:4317
processors:
batch:
timeout: 1s
send_batch_size: 50
exporters:
awsxray:
region: 'your-region'
awsemf:
region: 'your-region'
namespace: 'your-namespace'
log_group_name: '/aws/otel/traces'
log_stream_name: 'your-log-stream'
dimension_rollup_option: 'NoDimensionRollup'
service:
telemetry:
logs:
level: debug
pipelines:
traces:
receivers: [otlp]
processors: [batch]
exporters: [awsxray]
metrics:
receivers: [otlp]
processors: [batch]
exporters: [awsemf]
- Receivers: The OTLP receiver is configured to accept HTTP and gRPC protocols on ports 4318 and 4317, respectively.
- Processors: A batch processor controls how traces are collected and sent.
- Exporters: Trace data is exported to AWS X-Ray (
awsxray
exporter) and CloudWatch (awsemf
exporter).
To run the OpenTelemetry Collector using Docker:
docker run --rm -it \
-v $(pwd)/otel-collector-config.yaml:/etc/otel-collector-config.yaml \
-p 4317:4317 \
-p 4318:4318 \
-e AWS_REGION=us-east-1 \
-e AWS_PROFILE=default \
-v ~/.aws:/home/aoc/.aws \
amazon/aws-otel-collector:latest \
--config /etc/otel-collector-config.yaml
Alternatively, AWS credentials can be passed using environment variables.
Step 2: Instrumenting a Next.js Application with OpenTelemetry
To capture trace data from a Next.js application, OpenTelemetry must be integrated. The OTLPTraceExporter
is configured to export trace data over HTTP.
import { OTLPTraceExporter } from "@opentelemetry/exporter-trace-otlp-http";
import {
AlwaysOnSampler,
BatchSpanProcessor,
TraceIdRatioBasedSampler,
} from "@opentelemetry/sdk-trace-node";
import { registerOTel } from "@vercel/otel";
export function register() {
const otlpExporter = new OTLPTraceExporter({
url: process.env.OTEL_EXPORTER_OTLP_TRACES_ENDPOINT,
});
registerOTel({
serviceName: "your-service-name",
traceSampler:
process.env.NODE_ENV === "development"
? new AlwaysOnSampler()
: new TraceIdRatioBasedSampler(0.1),
spanProcessors: [new BatchSpanProcessor(otlpExporter)],
});
}
- OTLPTraceExporter: Configured to send traces to the OpenTelemetry Collector endpoint.
- Trace Sampler: Used an AlwaysOnSampler in development and a 10% sampling rate in production.
- BatchSpanProcessor: Batched trace data before sending it to the exporter.
Read More: Secure HTTP requests with security headers using Lambda@Edge and CloudFront
Step 3: Enabling Automatic Request Tracing in Next.js
In Next.js 13.4+, OpenTelemetry tracing can be automatically enabled for requests through auto-instrumentation. This removes the need to create a span for every request manually.
To enable auto-instrumentation in Next.js 13.4 and 14, the following configuration was added to the
file: next.config.js
module.exports = {
experimental: {
instrumentationHook: true,
},
};
For Next.js 15+, auto-instrumentation is enabled by default.
Also Read: Integrating OpenTelemetry with FastAPI in Python For Observability
Benefits After Implementation
After implementing OpenTelemetry with AWS X-Ray, the application now benefits from:
- Improved Observability: The entire request flow can be visualized in AWS X-Ray, making it easier to identify slow requests and bottlenecks.
- Faster Debugging: Instead of manually analyzing logs, tracing requests across services provides clear insights into performance issues.
- Enhanced Performance Optimization: Real-time metrics help optimize the application by addressing latency and inefficiencies.
- Seamless Auto-Instrumentation: Next.js 13.4+ simplifies tracing, reducing the need for manual span creation.
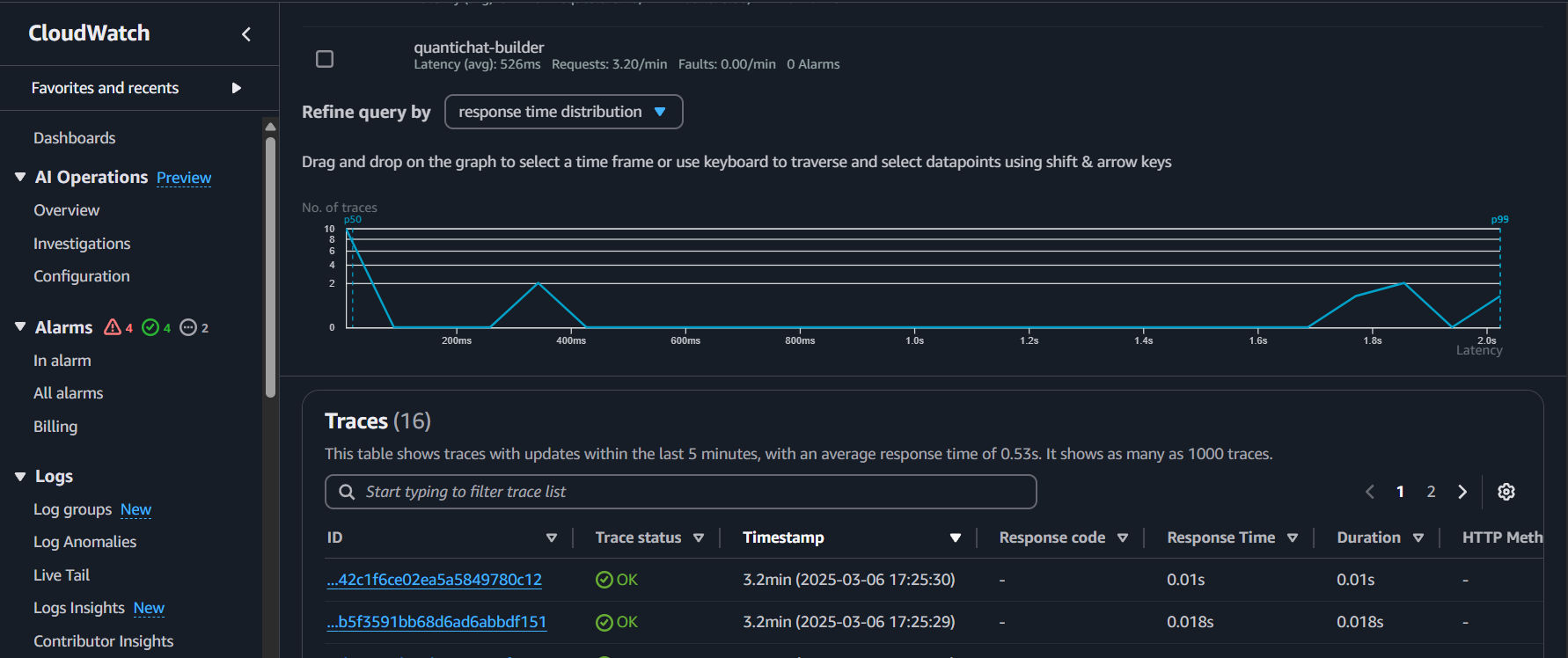
Conclusion
By integrating OpenTelemetry with AWS X-Ray, observability in the Next.js application has significantly improved. With trace data flowing seamlessly to AWS, monitoring requests, detecting errors, and optimizing performance have become more efficient.
Integrating OpenTelemetry with AWS X-Ray offers developers who want to enhance the observability of their distributed systems robust real-time tracing and performance insights.
Happy Tracing!
Read More:
- DevOps Outsourcing: How it works, benefits & how to get started
- What is DevOps Automation, and How does DevOps as a Service work?
- Top DevOps Security Tools For Protecting Your Development In 2025
- FinOps in AWS: Best Tools and Practices for Cloud Cost Optimization
- Manage Patching with AWS Systems Manager Custom Patch Baselines
- Build Serverless Payment Processing System with AWS Lambda, SQS, and Step Functions